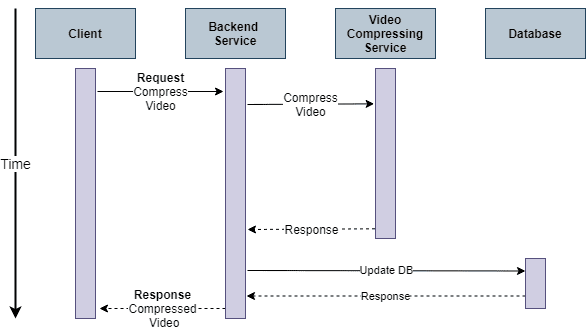
Context and Problem
In modern cloud computing and distributed systems, applications often need to perform long-running operations. These operations can range from data processing tasks to complex computations that take significant time to complete. In a synchronous request-reply model, the client must wait for the server to complete the operation and return a response, which can lead to inefficiencies and poor user experience. This is particularly problematic when the client needs to remain responsive and handle other tasks concurrently1.
Issues and Considerations
- Latency: Long-running operations can cause significant delays, making the client unresponsive.
- Scalability: Synchronous operations can limit the scalability of the system, as resources are tied up waiting for responses.
- Resource Utilization: Inefficient use of resources as threads or processes are blocked waiting for operations to complete.
- Error Handling: Managing errors and retries in a synchronous model can be complex and cumbersome.
- User Experience: Poor user experience due to delays and unresponsiveness2.
Solution
The Asynchronous Request-Reply pattern addresses these issues by decoupling the request and response processes. In this pattern, the client sends a request to the server and continues with other tasks without waiting for an immediate response. The server processes the request asynchronously and sends a reply once the operation is complete. This approach enhances efficiency, scalability, and user experience by allowing the client to remain responsive and handle other tasks concurrently1.
When to Use the Pattern
- Long-Running Operations: When operations take a significant amount of time to complete, such as data processing, file uploads, or complex computations.
- Microservices Architecture: In microservices architectures where services need to communicate asynchronously to maintain loose coupling and scalability.
- Event-Driven Systems: In event-driven systems where actions are triggered by events and responses are processed asynchronously.
- Improving User Experience: When you need to keep the client application responsive and improve user experience by avoiding long wait times2.
Real-World Example
Consider an e-commerce platform where users can place orders. When a user places an order, the system needs to perform several tasks, such as validating the order, processing payment, updating inventory, and sending a confirmation email. These tasks can take time, and making the user wait for all these operations to complete would result in a poor user experience. Instead, the platform can use the Asynchronous Request-Reply pattern. The client sends the order request and immediately receives an acknowledgment that the order is being processed. The server processes the order asynchronously and sends a confirmation email once all tasks are complete2.
Sample Pseudo Code in Python
Here’s a simple pseudo code example to illustrate the Asynchronous Request-Reply pattern in Python:
import time
import threading
import queue
# Simulate a long-running operation
def long_running_operation(data, result_queue):
time.sleep(5) # Simulate time-consuming task
result = f"Processed {data}"
result_queue.put(result)
# Client function to send request and handle response asynchronously
def client_request(data):
result_queue = queue.Queue()
threading.Thread(target=long_running_operation, args=(data, result_queue)).start()
print("Request sent, processing asynchronously...")
# Simulate doing other tasks while waiting for the response
for i in range(3):
print("Client is doing other tasks...")
time.sleep(1)
# Get the result from the queue
result = result_queue.get()
print(f"Received response: {result}")
# Client code
if __name__ == "__main__":
client_request("Order123")
In this pseudo code, the client_request
function sends a request to process an order and continues with other tasks while the order is being processed asynchronously. The long_running_operation
function simulates a time-consuming task, and the result is communicated back to the client using a queue.
By using the Asynchronous Request-Reply pattern, you can improve the efficiency, scalability, and user experience of your cloud and distributed systems. This pattern allows the client to remain responsive and handle other tasks while waiting for the server to complete long-running operations.
1: Azure Architecture Center – Asynchronous Request-Reply Pattern 2: CodeWithStu’s Blog – Asynchronous Request-Reply
Feel free to ask if you have any questions or need further details!